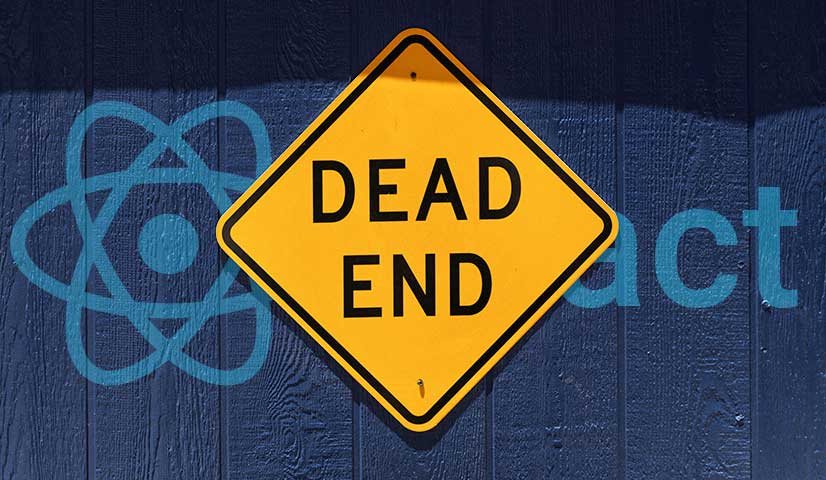
©Unsplash/Michal
Category: Thought
Is ReactJS a dead-end path?
React is like a modern celebrity - famous simply for being famous. It's often chosen as a safe solution, and no one can fault you for that. However, once you dive deeper, you'll be exposed to all its flaws. I predict that React will die out just as PHP did in the realm of HTML templating.
Tags: Technology.
React JS? No thank you, I'll go for modern frameworks.
I honestly believe working with ReactJS is a messy experience compared to other modern FE frameworks. It can be summarised as a waste of time. An extreme opinion? Allow me to share some arguments here. Let's start with a basic example, on a newbie level. To write a react functional (modern) component, containing just two input fields that are dynamically changing the line of text, you need that:import React, { useState } from 'react'; export default () => { const [a, setA] = useState(1); const [b, setB] = useState(2); function handleChangeA(event) { setA(+event.target.value); } function handleChangeB(event) { setB(+event.target.value); } return ( <div> <input type="number" value={a} onChange={handleChangeA}/> <input type="number" value={b} onChange={handleChangeB}/> <p>{a} + {b} = {a + b}</p> </div> ); };In VueJS, it will be simpler:
<template> <div> <input type="number" v-model.number="a"> <input type="number" v-model.number="b"> <p>{{a}} + {{b}} = {{a + b}}</p> </div> </template> <script> export default { data: function() { return { a: 1, b: 2 }; } }; </script>In Svelte (which you should be using if not doing it yet):
<script> let a = 1; let b = 2; </script> <input type="number" bind:value={a}> <input type="number" bind:value={b}> <p>{a} + {b} = {a + b}</p>Wow, React requires so much more code!
But let's talk about the next step on the learning curve. To get the knack of Redux, a state container commonly used with React, you're happy if you start now. With Redux-Toolkit it is quite straightforward. Unfortunately, your more experienced colleagues have used tones of different ways of doing it, including famous Redux-Saga or Thunk. What an effort needed to catch-up and be quick with all of that spaghetti. Please understand my point here, I'm not saying those libraries are bad, in fact some of them are brilliant. The problem is the entire mashed-up ecosystem, which costs you a lot of learning. In practice, the average developer has only 2-4 years of experience and is not productive in that environment. She/He consumes time paid by the employer to learn.
There are more surprises when you dig deeper. React is not written with Typescript but with Flow (Facebook attempts to make JS with type support). TS is added 'on top' by an external team. Which ends with unexpected results (check this).
I believe moving to simpler and cleaner frameworks is the path forward. They were created with all the experience gained while React was the leading edge. If you think about potential cons, there are only two, less documentation (examples) on the web, and the risk of those projects being abandoned.
As a last word, React is slow. Very slow.
Michal